MPI Best Practices for OLCF
Overview
The Message Passing Interface (MPI) implementation available on Frontier is the GPU-aware HPE Cray MPI library. Come spend an hour with us and Tim Mattox, from the HPE Center of Excellence, to learn best practices for getting the most out of MPI on Frontier. Among other things we will cover how to do efficient halo exchanges, how to diagnose MPI performance problems, how to avoid anti-patterns that hurt performance, and some background on why it is very important to post MPI receives before the messages arrive (which might sound backwards!).
Speaker:
Tim Mattox, Ph.D., HPC Performance Engineer, HPE Frontier Center of Excellence (COE)
Since 1993, Tim has worked on various aspects of HPC systems, including a 2.5 year stint as an Open MPI developer.
Who should Attend:
This training is open to all OLCF users.
Presentation:
(Slides | Recording)
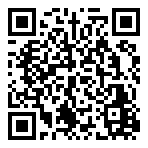